Stack and Tab Navigators
What is a Stack Navigator?
A Stack Navigator provides a way for your app to transition between screens where
each new screen is placed on top of a stack.
What is a Tab Navigator?
A Tab Navigator is a simple tab bar at the bottom of the screen that lets you
switch between different routes. Routes are lazily initialized -- their screen
components are not mounted until they are first focused.
In this article we will learn to use nested navigators in react native
by placing a stack navigator inside a tab navigator.
Setting Up
yarn add @react-navigation/native @react-navigation/bottom-tabs @react-navigation/stack
We want to create a navigation structure as follows:
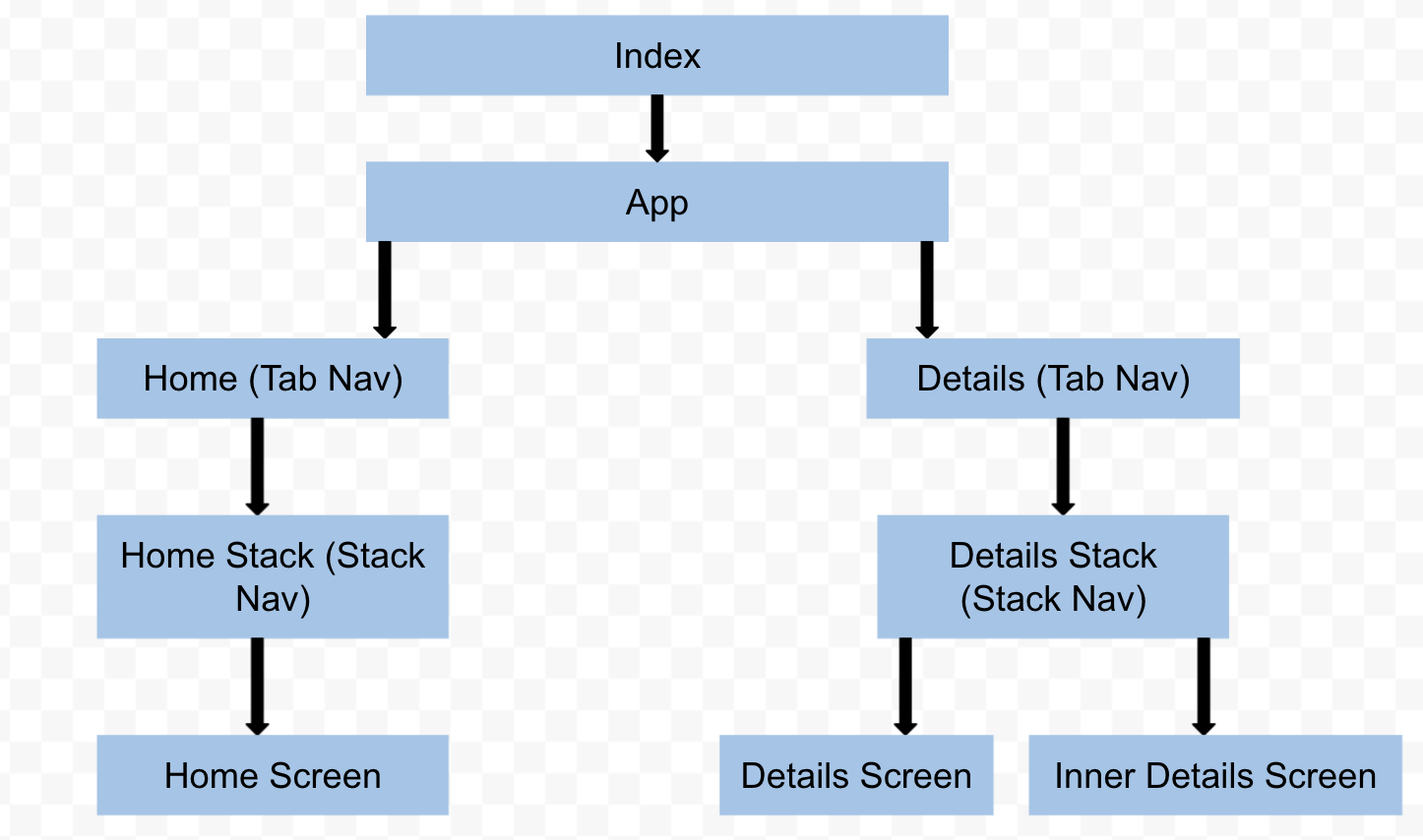
Create a file, src/navigation/Navigation.js,
where we will manage navigation for the app.
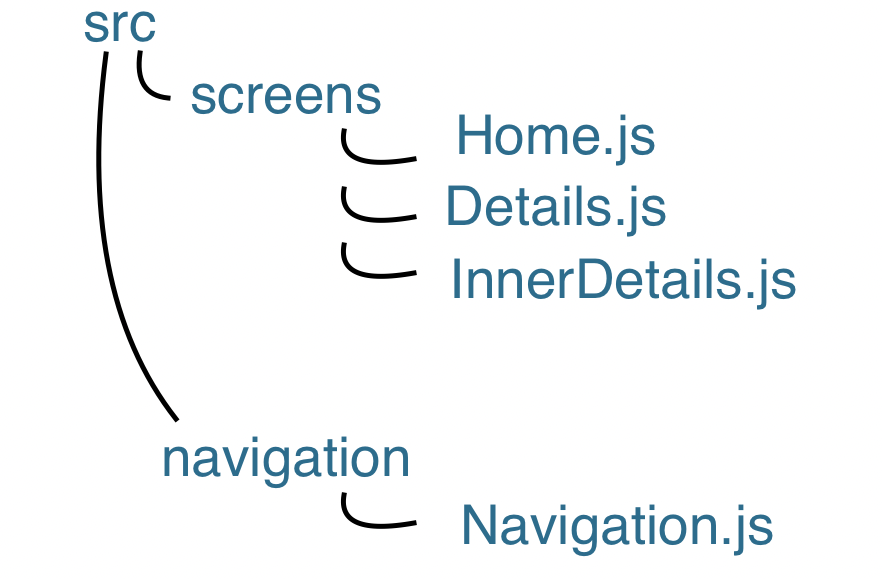
Building Stacks
Say I want to add tab icons for Home and Details in the Tab Navigator.
From the Details tab I should be able to navigate to an inner screen named
InnerDetails.js.
So we will need to create separate stacks using the Stack Navigator for the two
tabs that we want.
We want only one screen for the Home tab,
which is the loaded by default when the app starts.
The HomeStack would define that screen as follows:
Next we want a Details tab button
for navigating to the Details screen.
Inside that screen we want a button that
will display an inner screen named InnerDetails.js.
We will hide our Tab bar in this particular screen
with getFocusedRouteNameFromRoute
from @react-navigation/stack
.
With that in mind, the Details Stack would be as follows:
Configuring the Tab Bar
Now we will add the two tabs to the Tab bar.
We want the Home screen to be our landing page
so we should specify the HomeStack as the initial route in the Tab Navigator.
It is also possible to add styles to the Tab bar
for different orientations using the tabBarOptions
property of the Tab Navigator.
This can be useful for highlighting the active tab, for example,
as we will demonstrate shortly.
To know more about the props to customize the Tab navigator have a look at
https://reactnavigation.org/docs/bottom-tab-navigator/ .
The Tab bar with the Home and Details tabs would be as follows:
Putting it all together
And here is the final Navigation.js code in the Navigation file (src/navigation/Navigation.js):
The following image shows the output:
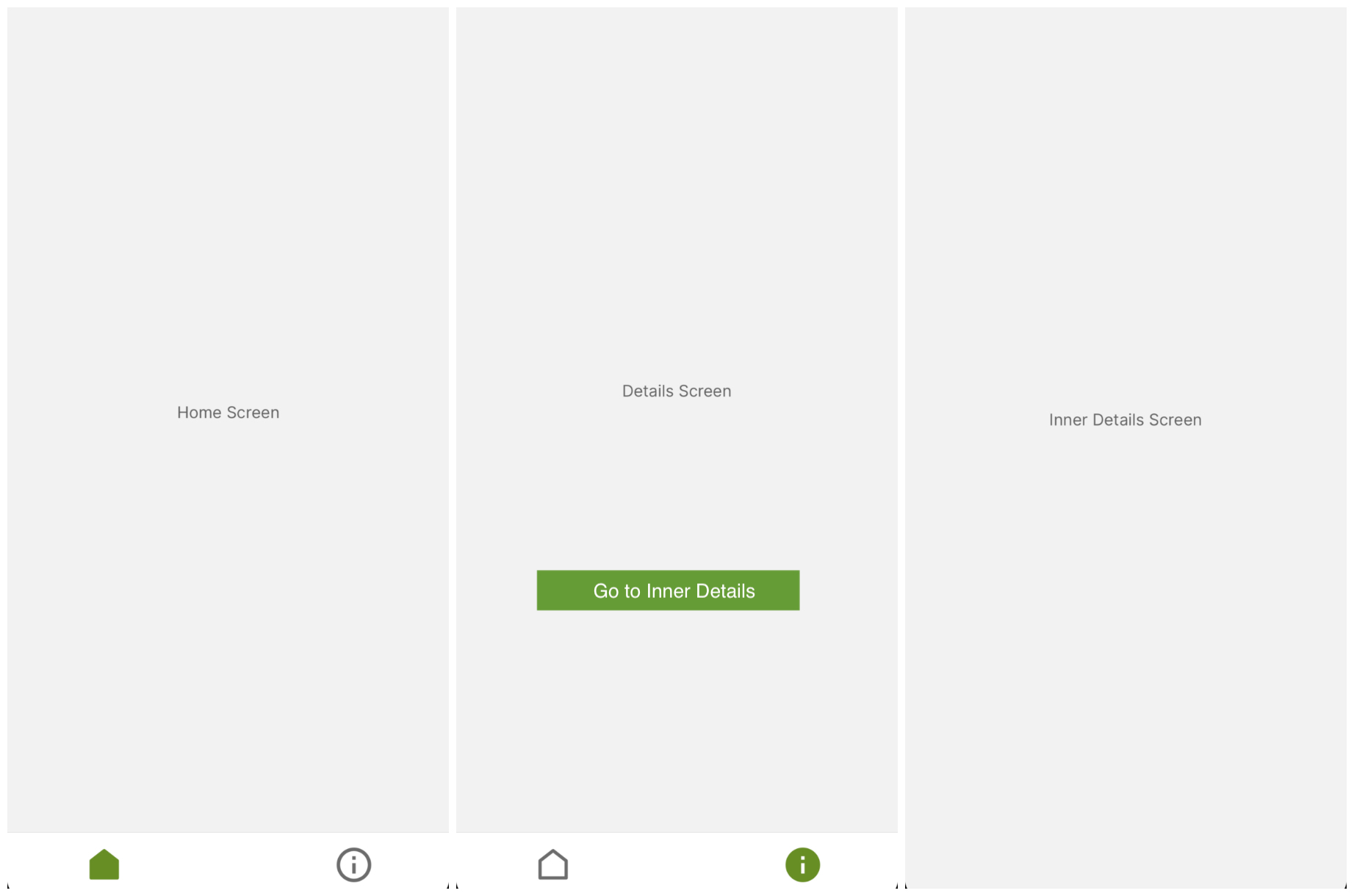